RichEditBox là một điều khiển chỉnh sửa văn bản đa dạng thức hỗ trợ văn bản được định dạng, siêu liên kết và nội dung phong phú khác. Các dự án WPF không hỗ trợ kiểm soát này. Vì vậy, nó sẽ được thực hiện trong Windows App. Sự kế thừa phân cấp của lớp RichEditBox như sau:
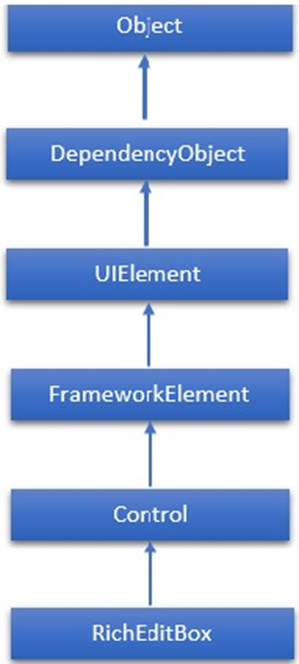
Đặc tính
Dưới đây là các thuộc tính được sử dụng phổ biến nhất của RichEditBox.
Sự kiện
Dưới đây là các sự kiện quan trọng và được sử dụng phổ biến nhất của RichEditBox.
Phương pháp
Dưới đây là các phương thức thường được sử dụng trong lớp RichEditBox.
Thí dụ
Ví dụ sau đây cho thấy cách mở và lưu tệp RTF trong RichEditBox. Đây là mã XAML để tạo và khởi tạo hai nút và một RichEditBox với một số thuộc tính và sự kiện.
<Page x:Class = "XAMLRichEditBox.MainPage"
xmlns = "http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x = "http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local = "using:XAMLRichEditBox"
xmlns:d = "http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc = "http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable = "d">
<Grid Background = "{ThemeResource ApplicationPageBackgroundThemeBrush}">
<Grid Margin = "120">
<Grid.RowDefinitions>
<RowDefinition Height="50"/>
<RowDefinition/>
</Grid.RowDefinitions>
<StackPanel Orientation = "Horizontal">
<Button Content = "Open file" Click = "OpenButton_Click"/>
<Button Content = "Save file" Click = "SaveButton_Click"/>
</StackPanel>
<RichEditBox x:Name = "editor" Grid.Row = "1"/>
</Grid>
</Grid>
</Page>
Đây là cách triển khai trong C # cho các sự kiện và xử lý tệp khác nhau
using System;
using System.Collections.Generic;
using System.IO; using System.Linq;
using System.Runtime.InteropServices.WindowsRuntime;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.Storage;
using Windows.Storage.Pickers;
using Windows.Storage.Provider;
using Windows.UI.ViewManagement;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
// The Blank Page item template is documented at
http://go.microsoft.com/fwlink/?LinkId=244438
namespace XAMLRichEditBox {
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
public sealed partial class MainPage : Page {
public MainPage() {
this.InitializeComponent();
}
private async void OpenButton_Click(object sender, RoutedEventArgs e) {
// Open a text file.
Windows.Storage.Pickers.FileOpenPicker open = new
Windows.Storage.Pickers.FileOpenPicker();
open.SuggestedStartLocation =
Windows.Storage.Pickers.PickerLocationId.DocumentsLibrary;
open.FileTypeFilter.Add(".rtf");
Windows.Storage.StorageFile file = await open.PickSingleFileAsync();
if (file != null) {
Windows.Storage.Streams.IRandomAccessStream randAccStream = await
file.OpenAsync(Windows.Storage.FileAccessMode.Read);
// Load the file into the Document property of the RichEditBox.
editor.Document.LoadFromStream(Windows.UI.Text.TextSetOptions.FormatRtf, randAccStream);
}
}
private async void SaveButton_Click(object sender, RoutedEventArgs e) {
if (((ApplicationView.Value != ApplicationViewState.Snapped) ||
ApplicationView.TryUnsnap())) {
FileSavePicker savePicker = new FileSavePicker();
savePicker.SuggestedStartLocation = PickerLocationId.DocumentsLibrary;
// Dropdown of file types the user can save the file as
savePicker.FileTypeChoices.Add("Rich Text", new List <string>() { ".rtf" });
// Default file name if the user does not type one in or select a file to replace
savePicker.SuggestedFileName = "New Document";
StorageFile file = await savePicker.PickSaveFileAsync();
if (file != null) {
// Prevent updates to the remote version of the file until we
//finish making changes and call
CompleteUpdatesAsync. CachedFileManager.DeferUpdates(file);
// write to file
Windows.Storage.Streams.IRandomAccessStream randAccStream = await
file.OpenAsync(Windows.Storage.FileAccessMode.ReadWrite);
editor.Document.SaveToStream(Windows.UI.Text.TextGetOptions.FormatRtf, randAccStream);
// Let Windows know that we're finished changing the file so the
// other app can update the remote version of the file.
FileUpdateStatus status = await CachedFileManager.CompleteUpdatesAsync(file);
if (status != FileUpdateStatus.Complete) {
Windows.UI.Popups.MessageDialog
errorBox = new Windows.UI.Popups.MessageDialog(
"File " + file.Name + " couldn't be saved.");
await errorBox.ShowAsync();
}
}
}
}
}
}
Khi bạn biên dịch và thực thi đoạn mã trên, nó sẽ tạo ra kết quả sau. Bạn có thể mở, chỉnh sửa và lưu bất kỳ tệp RTF nào trong ứng dụng này
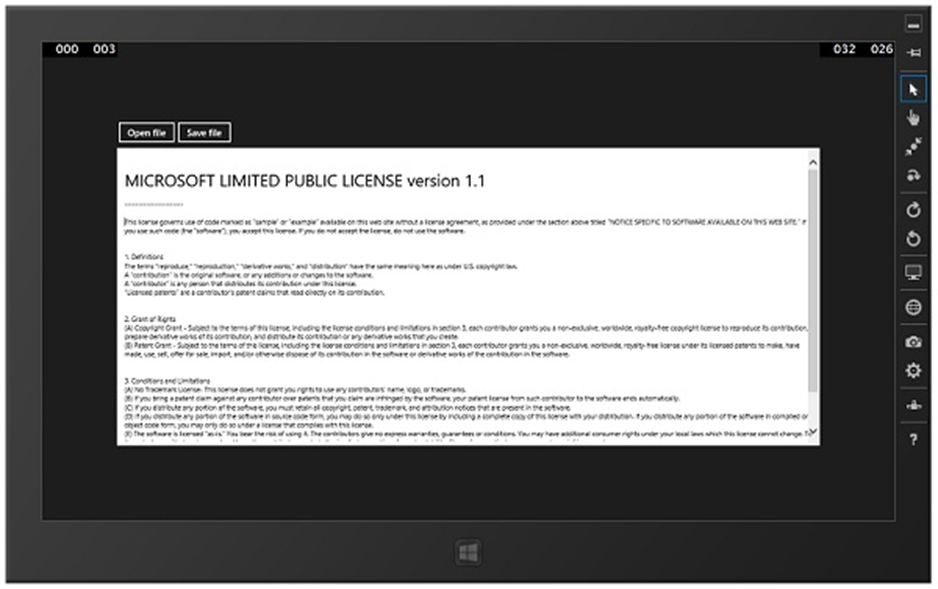
XAML – ScrollViewer
Điều khiển này cung cấp một vùng có thể cuộn được có thể chứa các phần tử hiển thị khác. Sự kế thừa phân cấp của lớp ScrollViewer như sau:
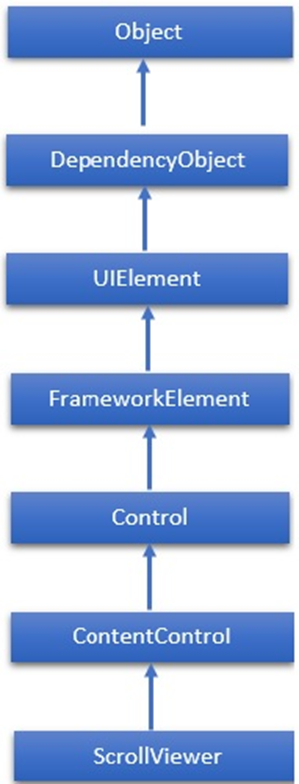
Đặc tính
Dưới đây là các thuộc tính thường được sử dụng của lớp ScrollViewer.
Sự kiện
Dưới đây là các sự kiện thường được sử dụng của lớp ScrollViewer.
Phương pháp
Dưới đây là các phương thức thường được sử dụng của lớp ScrollViewer.
Thí dụ
Ví dụ sau đây cho thấy cách thêm ScrollViewer trong ứng dụng XAML của bạn. Đây là mã XAML trong đó hai TextBlock được thêm vào và một với ScrollViewer và được khởi tạo với một số thuộc tính và sự kiện.
<Window x:Class = "XAMLScrollViewer.MainWindow"
xmlns = "http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x = "http://schemas.microsoft.com/winfx/2006/xaml"
Title = "MainWindow" Height = "550" Width = "604">
<Grid>
<StackPanel>
<!-- A large TextBlock. -->
<TextBlock Width = "300" TextWrapping = "Wrap" Margin = "0,0,0,30"
Text="Lorem ipsum dolor sit amet, consectetur adipiscing elit.
Sed ac mi ipsum. Phasellus vel malesuada mauris. Donec pharetra,
enim sit amet mattis tincidunt, felis nisi semper lectus, vel
porta diam nisi in augue. Pellentesque lacus tortor, aliquam et
faucibus id, rhoncus ut justo. Sed id lectus odio, eget pulvinardiam.
Suspendisse eleifend ornare libero, in luctus purus aliquet non.
Sed interdum, sem vitae rutrum rhoncus, felis ligula ultrices
sem, in eleifend eros ante id neque." />
<!-- The same large TextBlock, wrapped in a ScrollViewer. -->
<ScrollViewer Height = "200" Width = "200"
HorizontalScrollBarVisibility = "Auto"
VerticalScrollBarVisibility = "Auto">
<TextBlock Width = "300" TextWrapping = "Wrap"
Text = " This license governs use of code marked as “sample” or
“example” available on this web site without a license
agreement, as provided under the section above titled “NOTICE
SPECIFIC TO SOFTWARE AVAILABLE ON THIS WEB SITE.”
If you use such code (the “software”), you accept this license.
If you do not accept the license, do not use the software.
Lorem ipsum dolor sit amet, consectetur adipiscing elit.
Sed ac mi ipsum. Phasellus vel malesuada mauris.
Donec pharetra, enim sit amet mattis tincidunt, felis nisi semper lectus, vel porta diam nisi in augue.
Pellentesque lacus tortor, aliquam et faucibus id,
rhoncus ut justo. Sed id lectus odio, eget pulvinar diam.
Suspendisse eleifend ornare libero, in luctus purus aliquet non.
Sed interdum, sem vitae rutrum rhoncus,
felis ligula ultrices sem, in eleifend eros ante id neque." />
</ScrollViewer>
</StackPanel>
</Grid>
</Window>
Khi bạn biên dịch và thực thi đoạn mã trên, nó sẽ tạo ra kết quả sau:
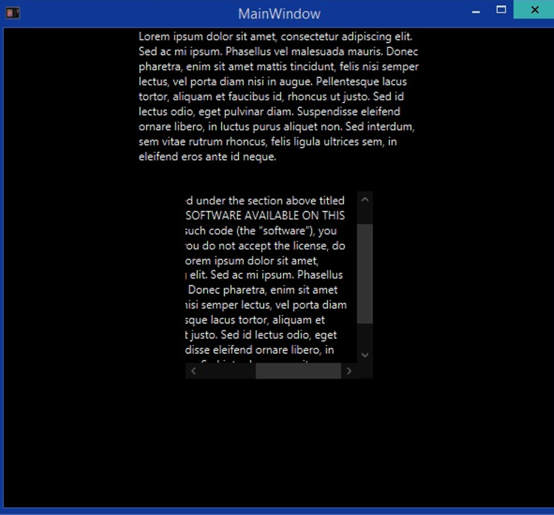